Mastering Python in 2025: A Comprehensive Guide with Tips and Insights
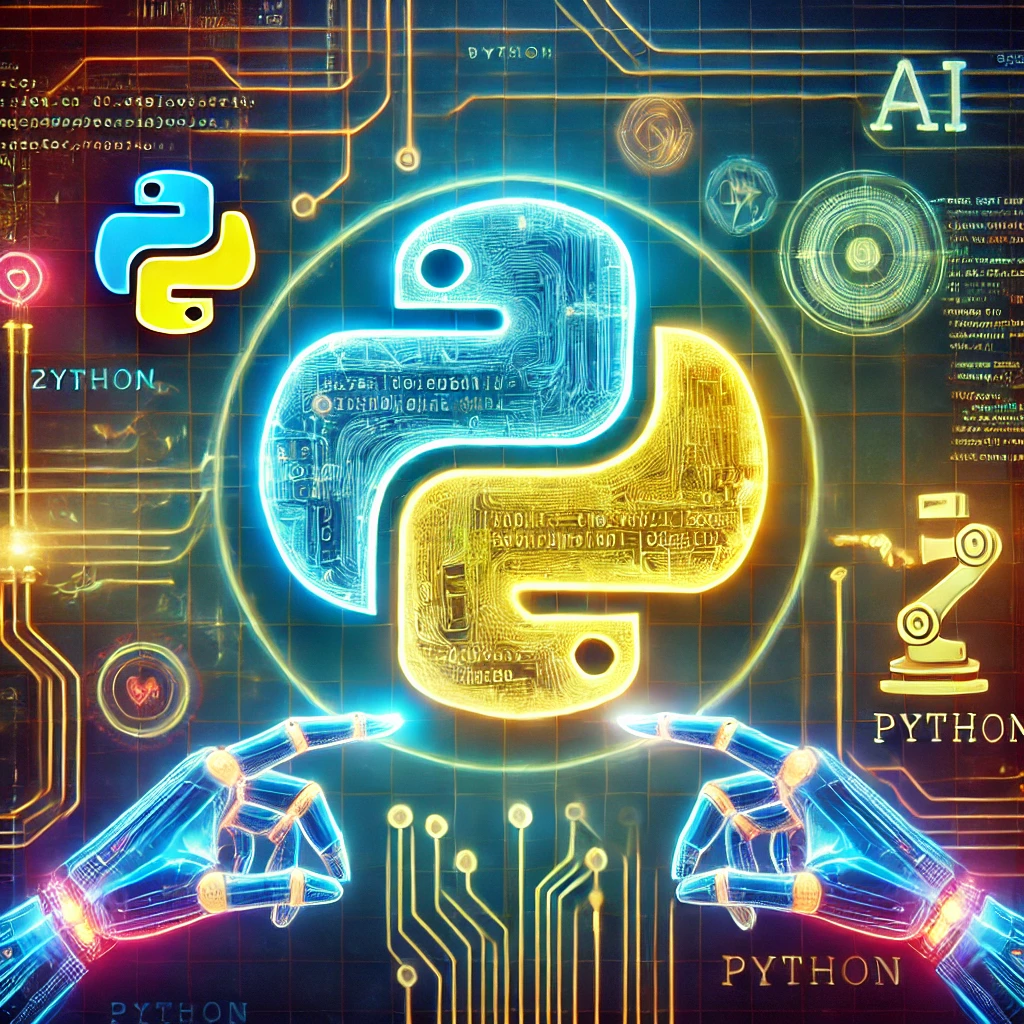
Python has become one of the most popular and versatile programming languages in the world. Whether you’re a beginner looking to start coding or an experienced developer exploring new possibilities, Python offers simplicity, flexibility, and an extensive ecosystem.
This guide provides an in-depth look into Python, from its basics to advanced applications, along with practical tips, structured headings, and bullet points to help you on your Python journey.
📖 What is Python?
Python is a high-level, interpreted programming language known for its easy-to-read syntax and vast library support. It is widely used in various domains, such as web development, data science, machine learning, artificial intelligence, and more.
💡 Why Learn Python in 2025?
- Demand Across Industries: Python is used in industries like finance, healthcare, gaming, and tech startups.
- Diverse Applications: From AI to web development, Python’s versatility makes it invaluable.
- Community Support: Python boasts one of the largest and most active developer communities.
🛠️ Getting Started with Python
1. Installing Python
- Download the latest version from Python.org.
- Use package managers like pip to install additional libraries.
2. Setting Up Your Environment
- Use IDEs like PyCharm, VS Code, or Jupyter Notebook for an efficient coding experience.
- Install virtual environments to manage dependencies:bash
pip install virtualenv virtualenv my_project source my_project/bin/activate
🔍 Python Basics
1. Variables and Data Types
Python supports several data types:
- Numeric:
int
,float
- Text:
str
- Boolean:
bool
- Collections:
list
,tuple
,set
,dict
Example:
name = "Python"
version = 3.10
is_popular = True
print(f"{name} version {version} is popular: {is_popular}")
2. Control Structures
- If-Else Statements:python
age = 18 if age >= 18: print("You're an adult.") else: print("You're a minor.")
- Loops:python
for i in range(5): print(i)
⚡ Intermediate Python Concepts
1. Functions
Organize your code into reusable blocks:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
2. File Handling
Read and write files:
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, Python!")
# Reading from a file
with open("example.txt", "r") as file:
print(file.read())
3. Object-Oriented Programming (OOP)
Use classes and objects:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} says Woof!"
dog = Dog("Buddy", "Golden Retriever")
print(dog.bark())
🚀 Advanced Python Topics
1. Libraries and Frameworks
- Web Development: Django, Flask
- Data Science: Pandas, NumPy, Matplotlib
- Machine Learning: TensorFlow, PyTorch, Scikit-learn
2. Decorators and Generators
- Decorators: Add functionality to existing functions.python
def decorator(func): def wrapper(): print("Before function call") func() print("After function call") return wrapper @decorator def say_hello(): print("Hello!") say_hello()
- Generators: Yield values one at a time, saving memory.python
def generate_numbers(): for i in range(5): yield i for num in generate_numbers(): print(num)
3. Asynchronous Programming
Handle multiple tasks concurrently:
import asyncio
async def say_hello():
await asyncio.sleep(1)
print("Hello!")
async def main():
await asyncio.gather(say_hello(), say_hello())
asyncio.run(main())
🔑 Tips for Mastering Python
- Practice Daily: Consistent practice is key to mastering Python.
- Contribute to Open Source: Join GitHub projects to enhance your skills.
- Explore Libraries: Familiarize yourself with Python libraries relevant to your field.
- Build Projects: Work on projects like:
- A personal portfolio website
- A simple chatbot
- A data visualization dashboard
- Join Communities: Engage with communities like Stack Overflow, Reddit, and Python Discord.
- Stay Updated: Follow Python blogs, YouTube channels, and newsletters for the latest trends.
🎯 Python Use Cases in 2025
- Data Analysis: Analyze and visualize complex datasets using Pandas and Matplotlib.
- AI and ML: Develop AI models with TensorFlow or PyTorch.
- Web Applications: Build dynamic websites with Flask or Django.
- Automation: Automate repetitive tasks using scripts.
📘 Resources to Learn Python
- Free Online Platforms:
- Codecademy
- Python.org tutorials
- Books:
- “Automate the Boring Stuff with Python”
- “Python Crash Course”
- YouTube Channels:
- Corey Schafer
- Programming with Mosh
🏁 Conclusion
Python remains a powerful and in-demand language, offering endless possibilities in technology and beyond. Whether you’re diving into web development, exploring AI, or simply automating daily tasks, Python equips you with the tools to succeed.
Start coding today and unlock the power of Python in 2025! 🚀
What’s Your Next Python Project?
Share your Python goals or favorite tips in the comments below. Let’s inspire each other to grow! 😊
Algorithms Artificial Intelligence Artificial Intelligence and Machine Learning Basics of Computer Science Best AI Tools Blogging Career Roadmap ChatGPT Coding Skills Computer Science Computer Science Concepts Computer Science for Beginners Content Creation Data Science Data Structures Free Online Courses HR technology Insight blogs Introduction to Computer Science Machine Learning Python Programming Recruitment automation Social Media Software Engineering
- Artificial
- Intelligence
- MACHINE LEARNING
- Web Devlopments
- CODING AND PROGRAMMING
- Prompt
- Engineering
- DIGITAL MARKETING
- SQL
- Graphic Design
- PYTHON
- generative ai
- Data SCIENCE
Recent Posts
-
Understanding Artificial Intelligence: A Beginner’s Guide to AI | Simplified Computer Science7 October 2024/0 Comments
-
Effective Project Execution: From Inception to Completion9 September 2024/
-
How to Create a Project Website on WordPress Without Coding9 September 2024/
M | T | W | T | F | S | S |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |